Our experts love to share knowledge. And it shows! Whether you have a business or technology need, you've come to the right place to find information you can use.
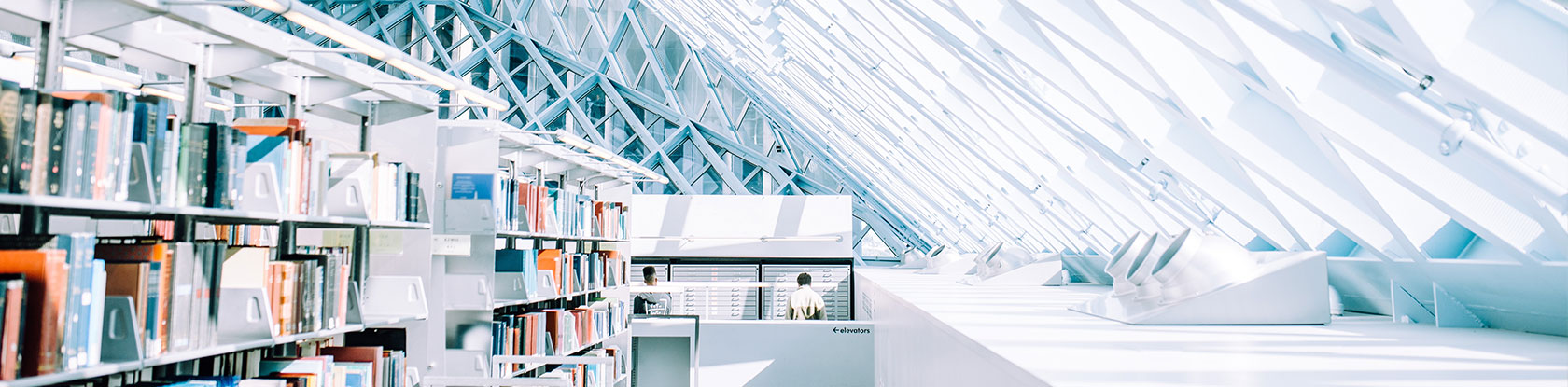
Our Blog
Filter By Topic
Filter By Industry
Our experts love to share knowledge. And it shows! Whether you have a business or technology need, you've come to the right place to find information you can use.